Random numbers have a wide range of applications all across programming. Testing for functionality is a big part of it. Generating unique IDs, passwords, or other random strings of characters is another big use case. There are several ways of generating random numbers in Bash, and today we will check them out.
Generating random numbers in Bash
Using the shuf command
The shuf command can be used to create permutations of random characters. Here, we will only see the option to generate a random integer. If you need to generate between A and B, and C number of numbers are required, the command becomes:
shuf -i A-B -nC
So, for example, generating three numbers between 10 and 40:
shuf -i 10-40 -n3
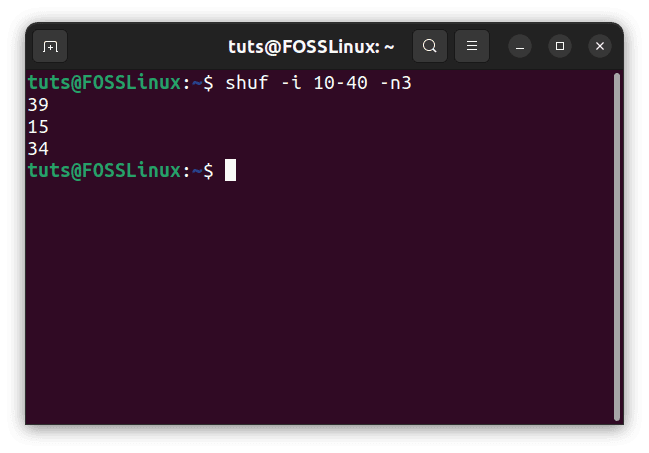
Using /dev/urandom
As we look into other methods, we get deeper into the working of Linux. The /dev/urandom file is a file that keeps collects random environmental noise from device drivers and creates an entropy pool, which is used to create random numbers. Since this is not a normal file, it needs to be used through some other commands to be read. We will use the od command, which is used to dump files in different formats. The ranging method is quite different in this case, though. It works on the number of bytes:
od /dev/urandom -A n -t d -N 1
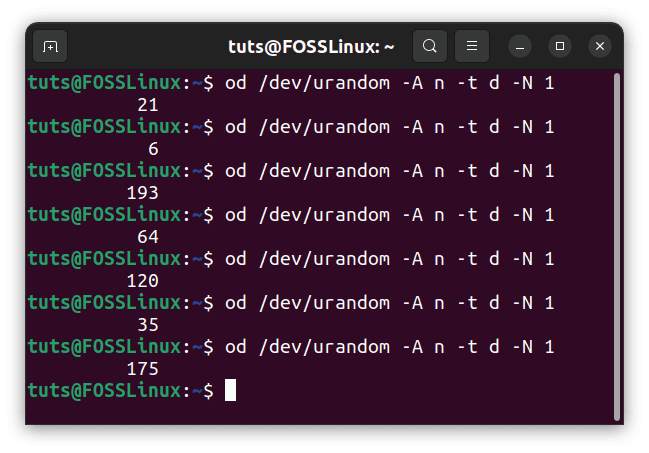
This command will generate a number that will not exceed 255 because that is the maximum size of one byte.
Using the $RANDOM variable
The most direct way that Bash provides to generate a random number is the $RANDOM variable. Unlike most variables with a constant value, and as the name suggests, echoing this variable gives a random number between 0 and 32767:
echo $RANDOM
But the issue is that, more often than not, we need a random number within a specific range. There is a very clever workaround to make this variable work that way. Say you want the number to be between A and B; the command would look like this:
echo $[ $[ $RANDOM % $[ B-A+1] ] + A ]
To break this down, let us take an example. Say we want a random number between 10 and 40. We can think of it another way: generate a random number between 0 and 30 and add 10. Hence, if we generate a random number in the range spanned by the two required boundaries (here, 40-10 = 30) and add it to the lower bound (random number between 0 and 30, but shifted 10 places higher), we can get a random number in the range we want.
How do you get a random number between 0 and some number? That is relatively easier. We use the remainder. Here, we want a number between 0 and 30. For whatever random number is generated, if we calculate its remainder with 30, we will get a number between 0 and 29. But wait, we want a number between 0 and 30. So what if we calculate the remainder with 31? That way, we get a proper range between 0 and 30.
So finally, we divide the random number by the range spanned by the boundary numbers + 1, and we shift it up by the lower boundary. All in all, it gives the formula mentioned above. So in our case of a random number between 10 and 40, the command becomes:
echo $[ $[ $RANDOM % $[ 40-10+1] ] + 10 ]
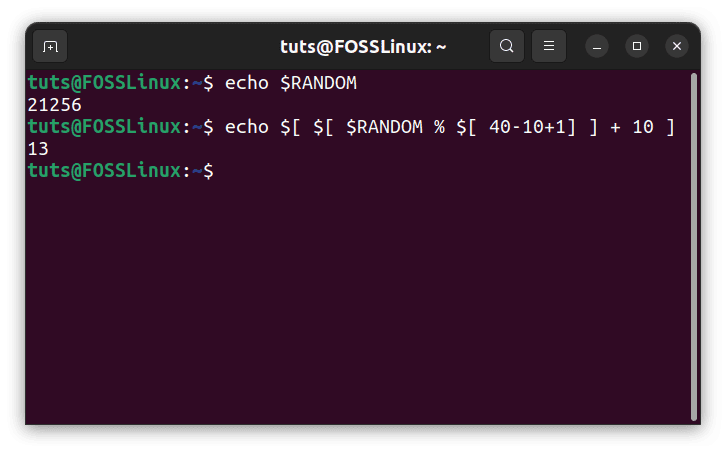
Since %RANDOM is only a variable, there isn’t much more to explore. It is just a number, so your imagination is the limit with mathematical operations. Nevertheless, you can mold it to suit whatever you have in mind.
Conclusion
As we have seen, there are several ways of creating random numbers in Bash. Some are more ubiquitous than the rest, and some are simpler than the rest. In any case, Bash will have you covered for whatever requirement you might have. We hope this article was helpful to you. Cheers!